Commits on Source (2)
-
csteindl authored64e5cdac
-
81a0d98e
print_materials/5_region_segmentation.pdf
0 → 100644
File added
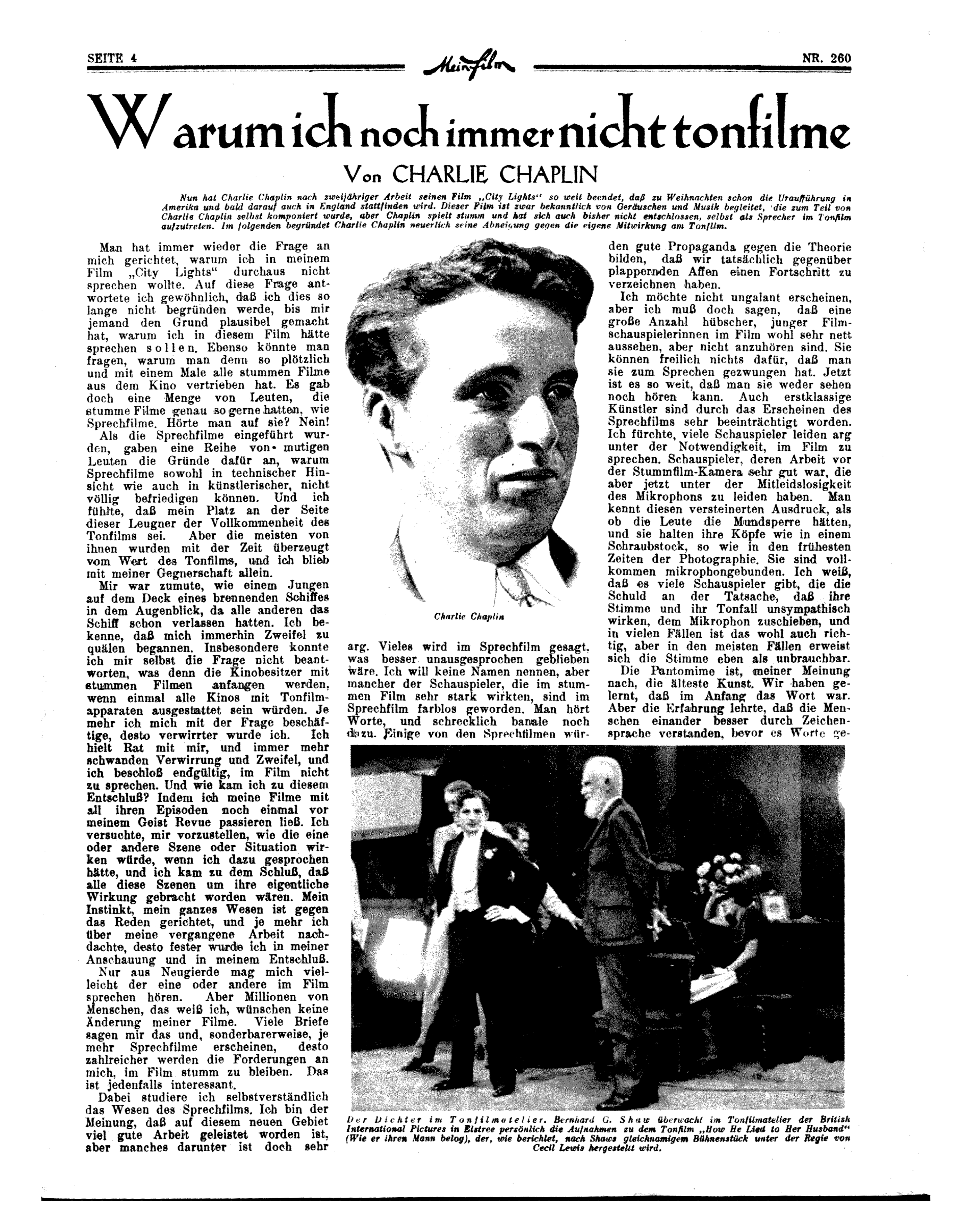
| W: | H:
| W: | H:
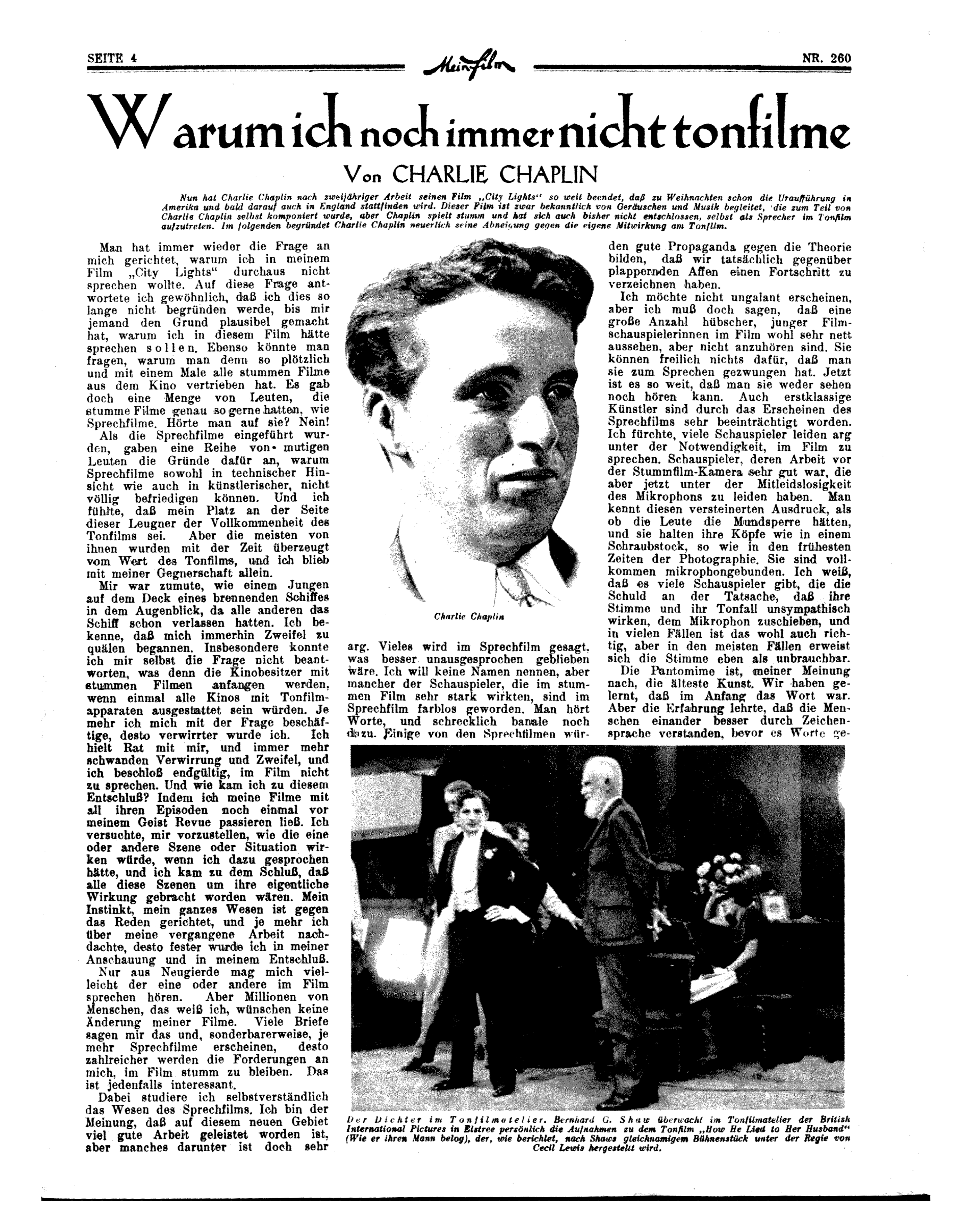
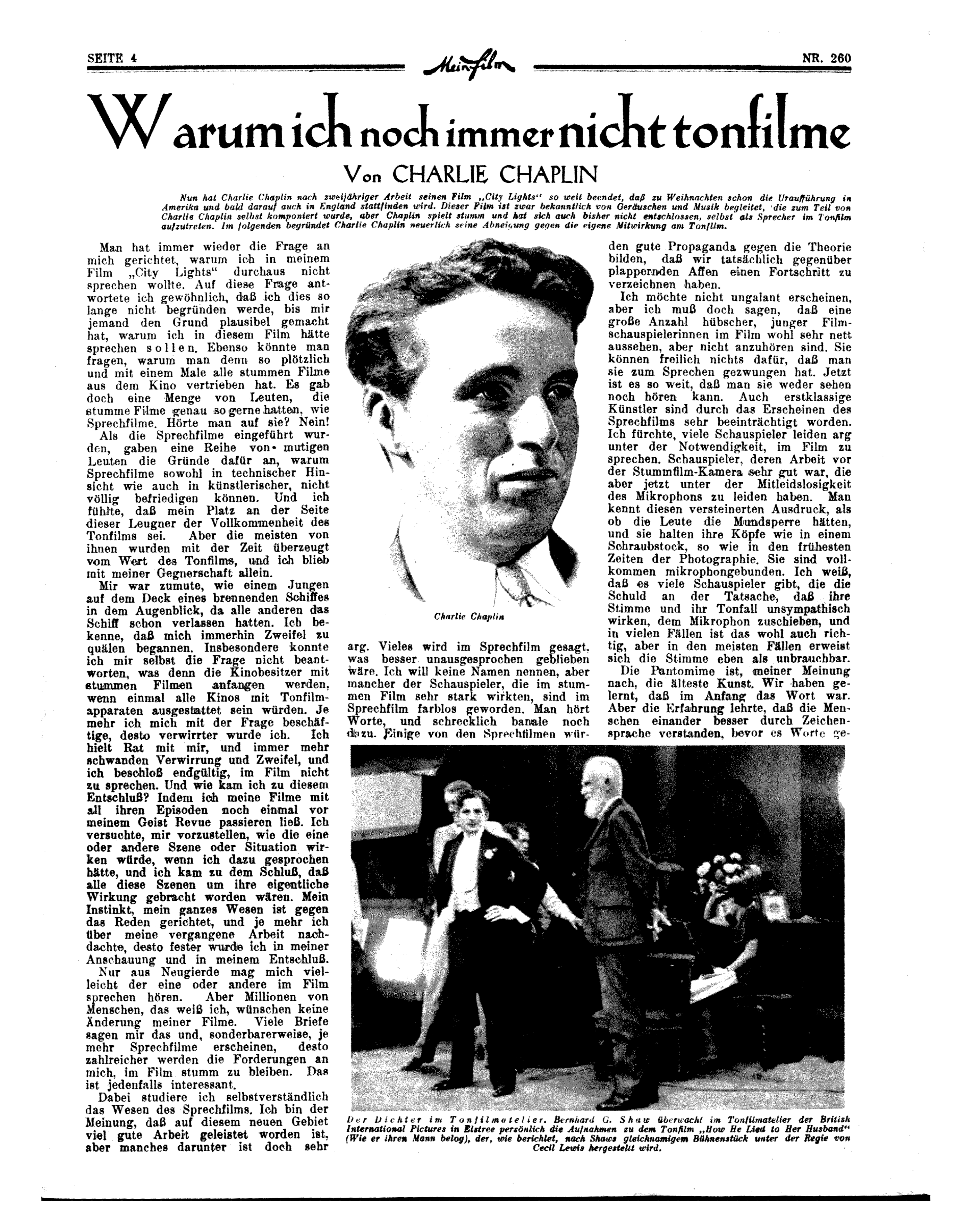
This diff is collapsed.
print_materials/6_line_detection.pdf
0 → 100644
File added
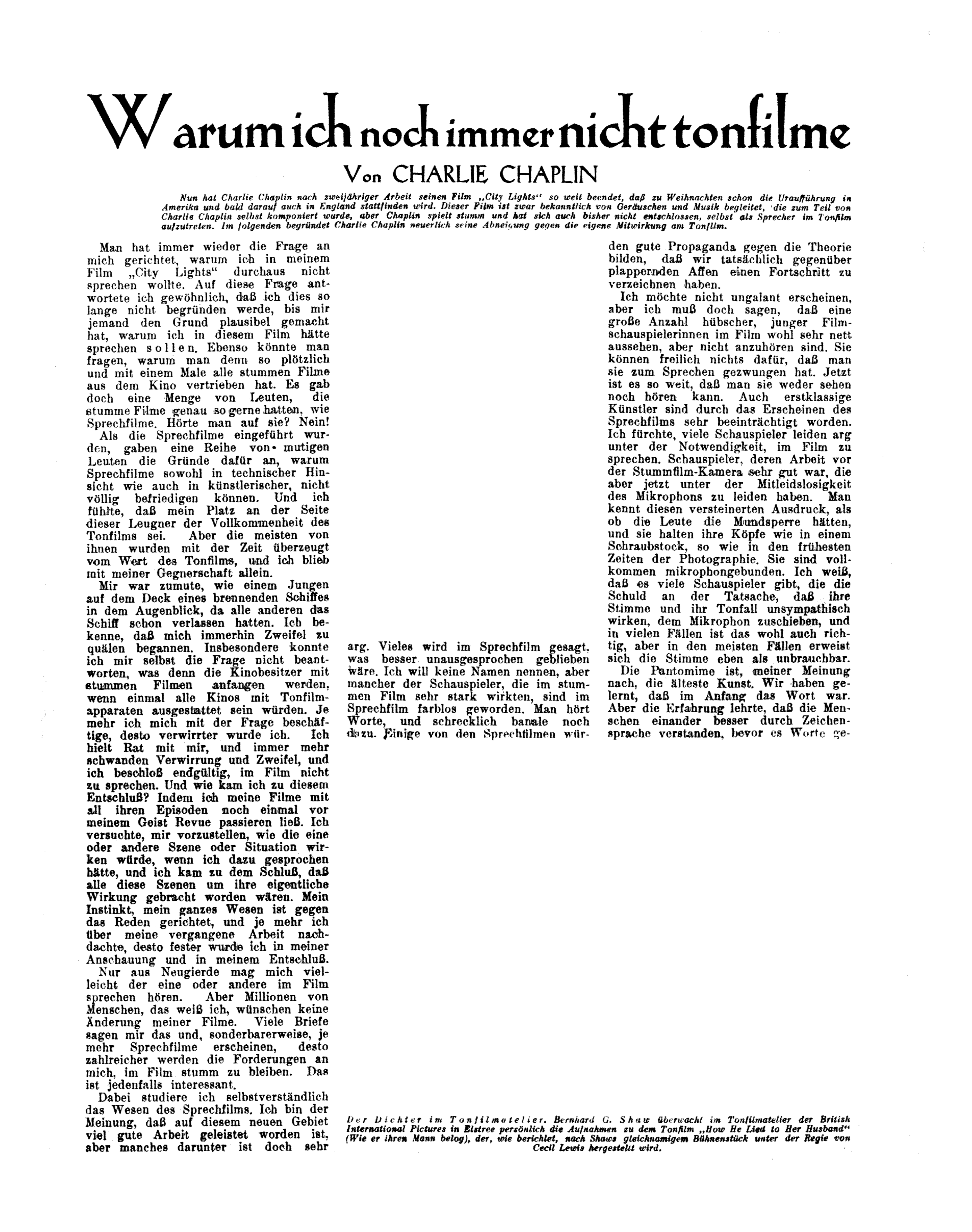
| W: | H:
| W: | H:
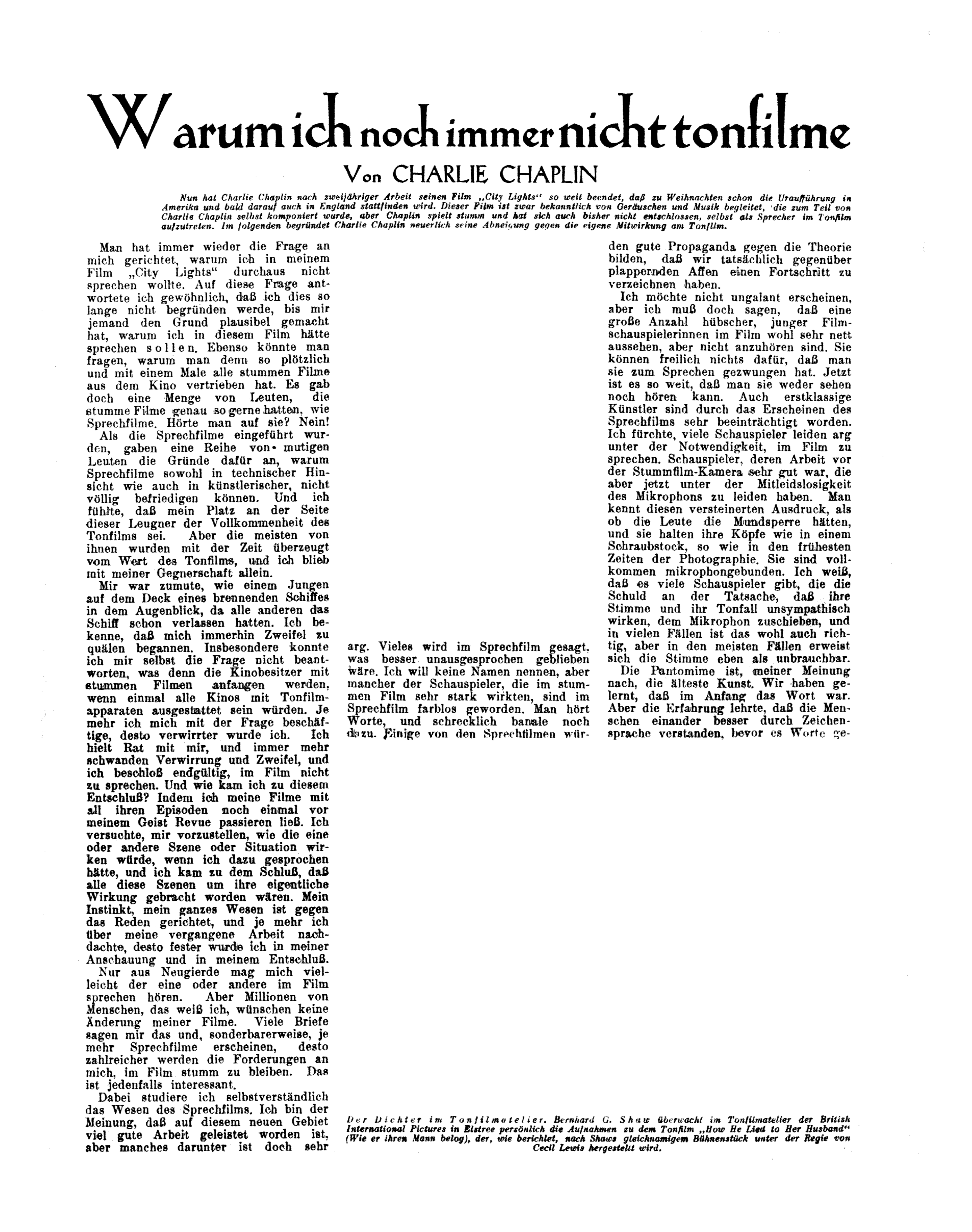
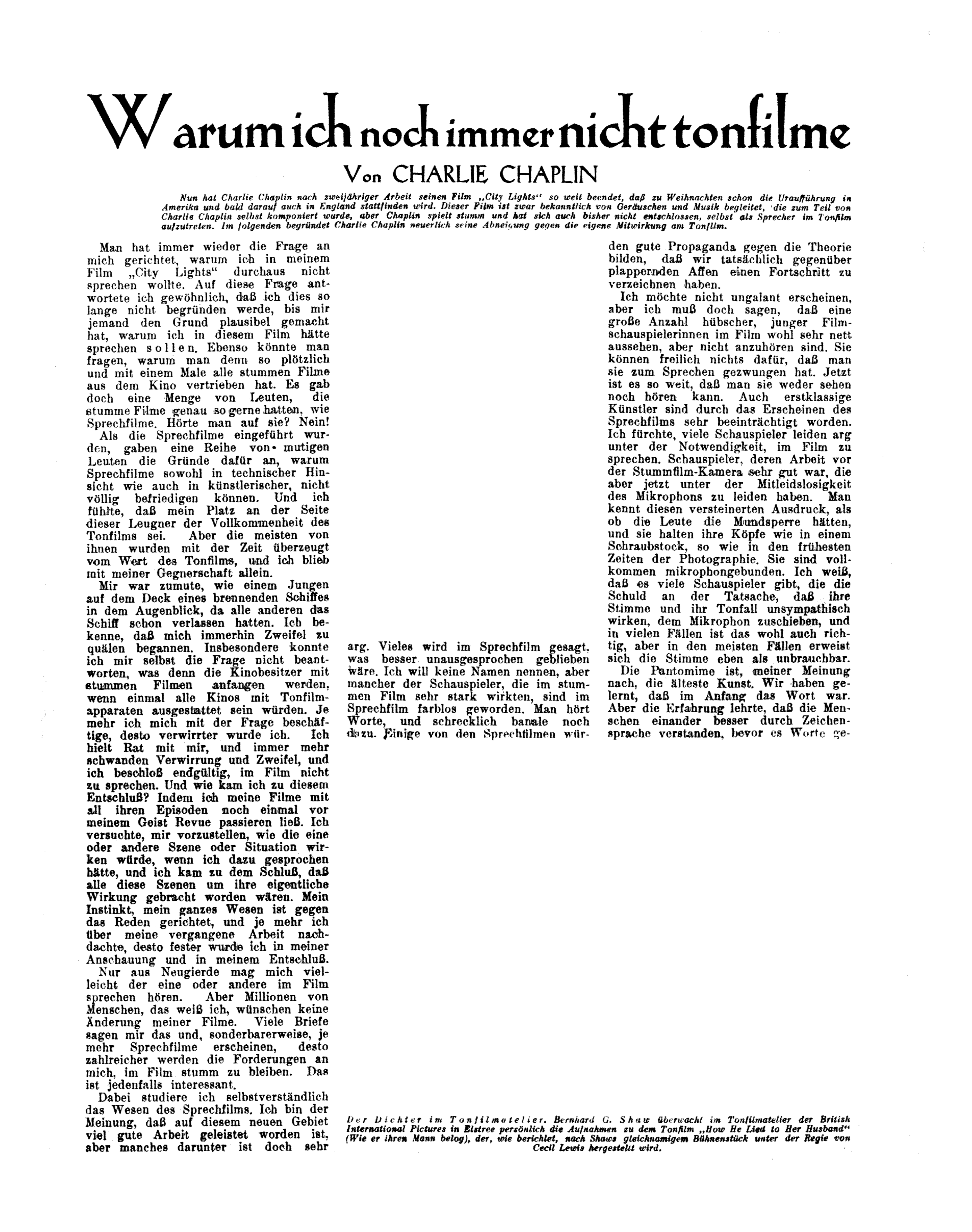
This diff is collapsed.
print_materials/7_word_detection.pdf
0 → 100644
File added
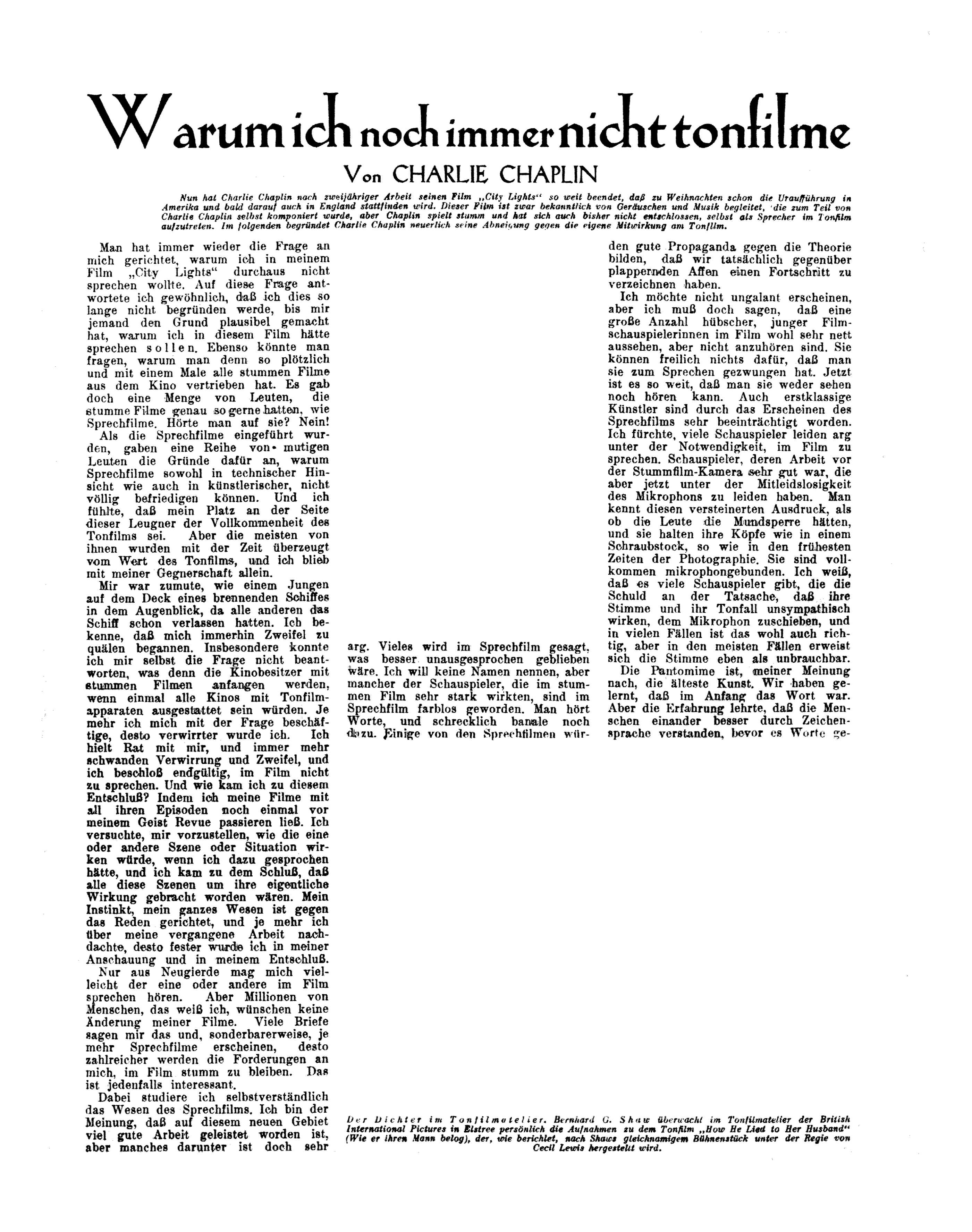
| W: | H:
| W: | H:
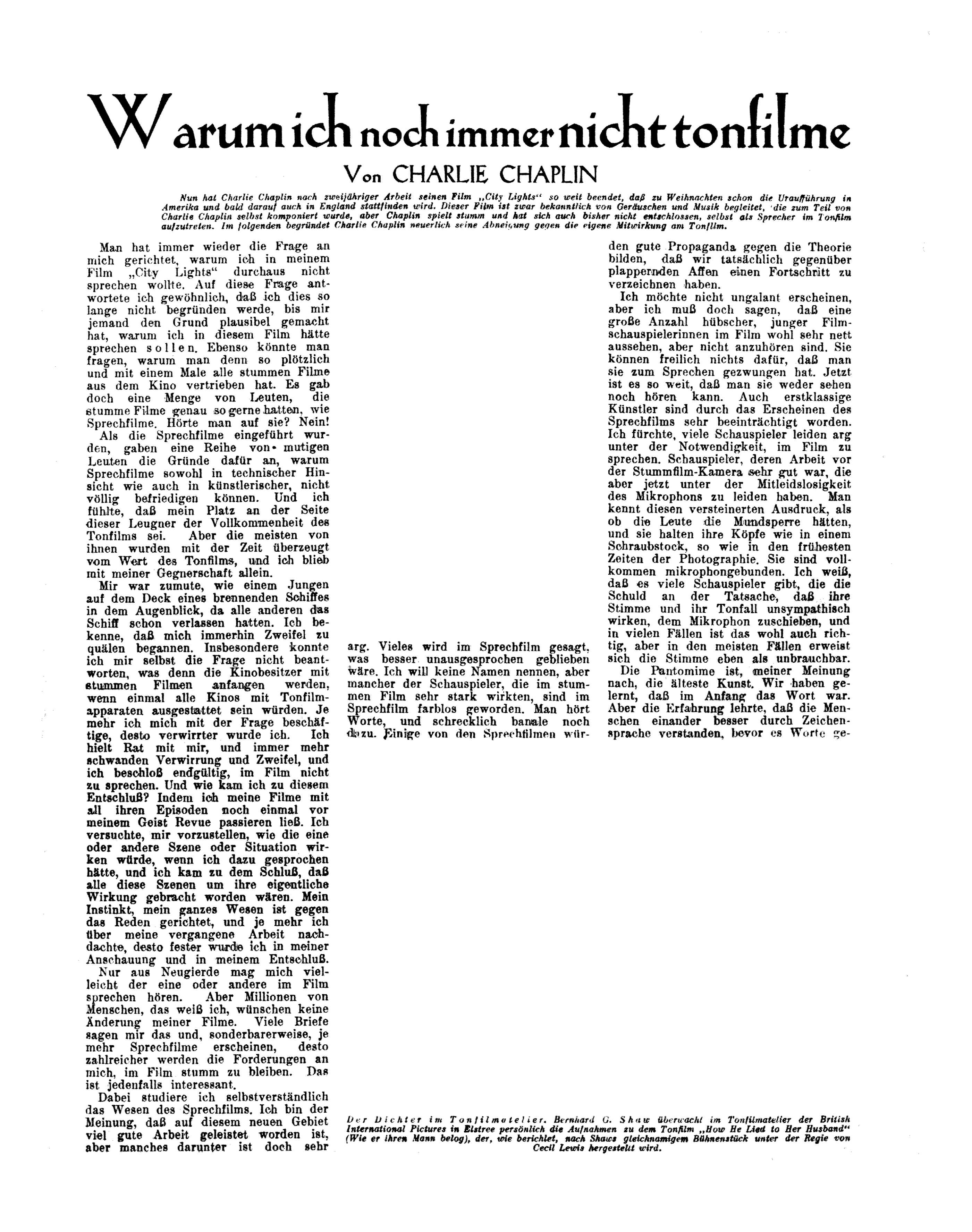
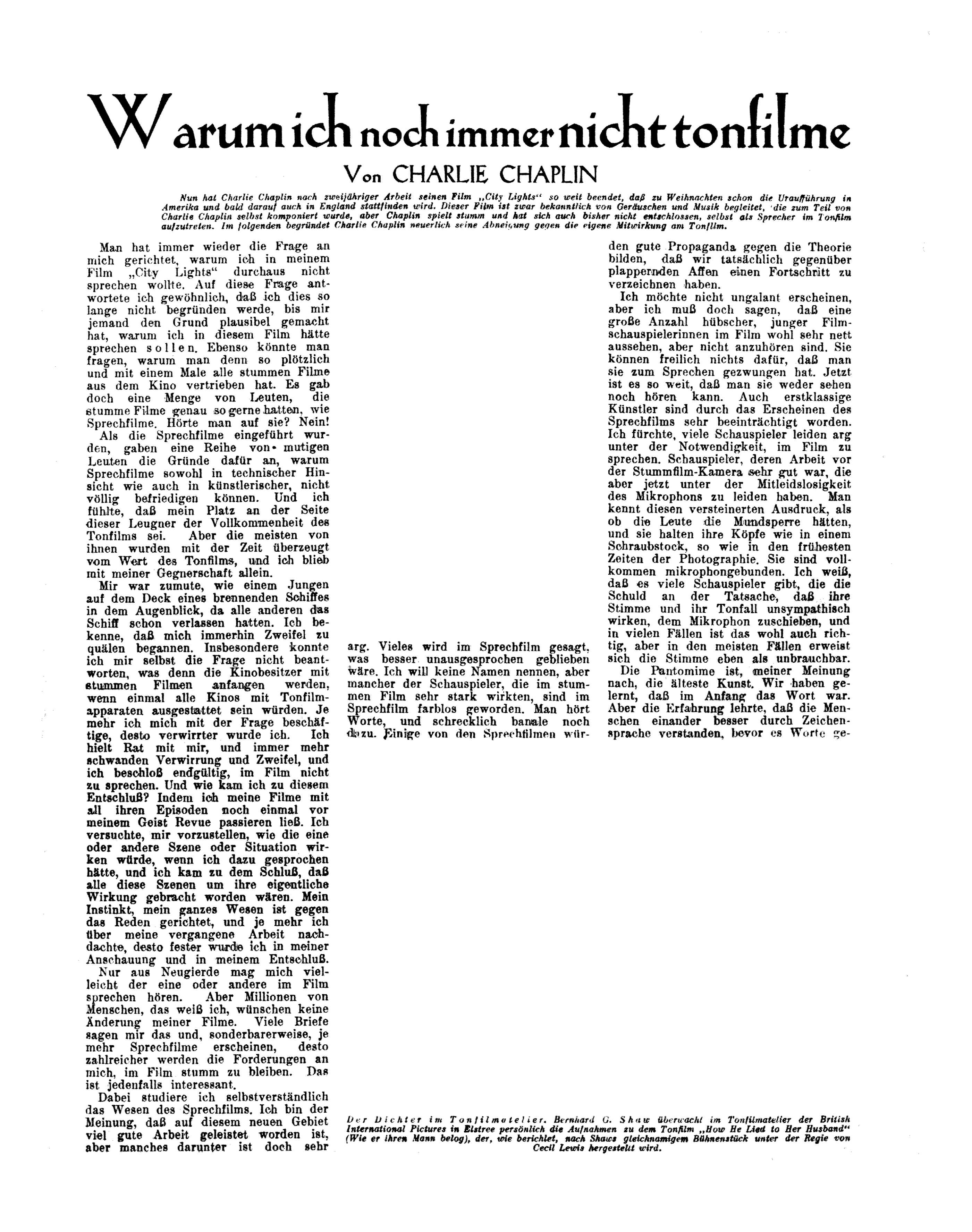
This diff is collapsed.
print_materials/ocr/draw_overlays.ipynb
0 → 100644
print_materials/ocr/requirements.txt
0 → 100644